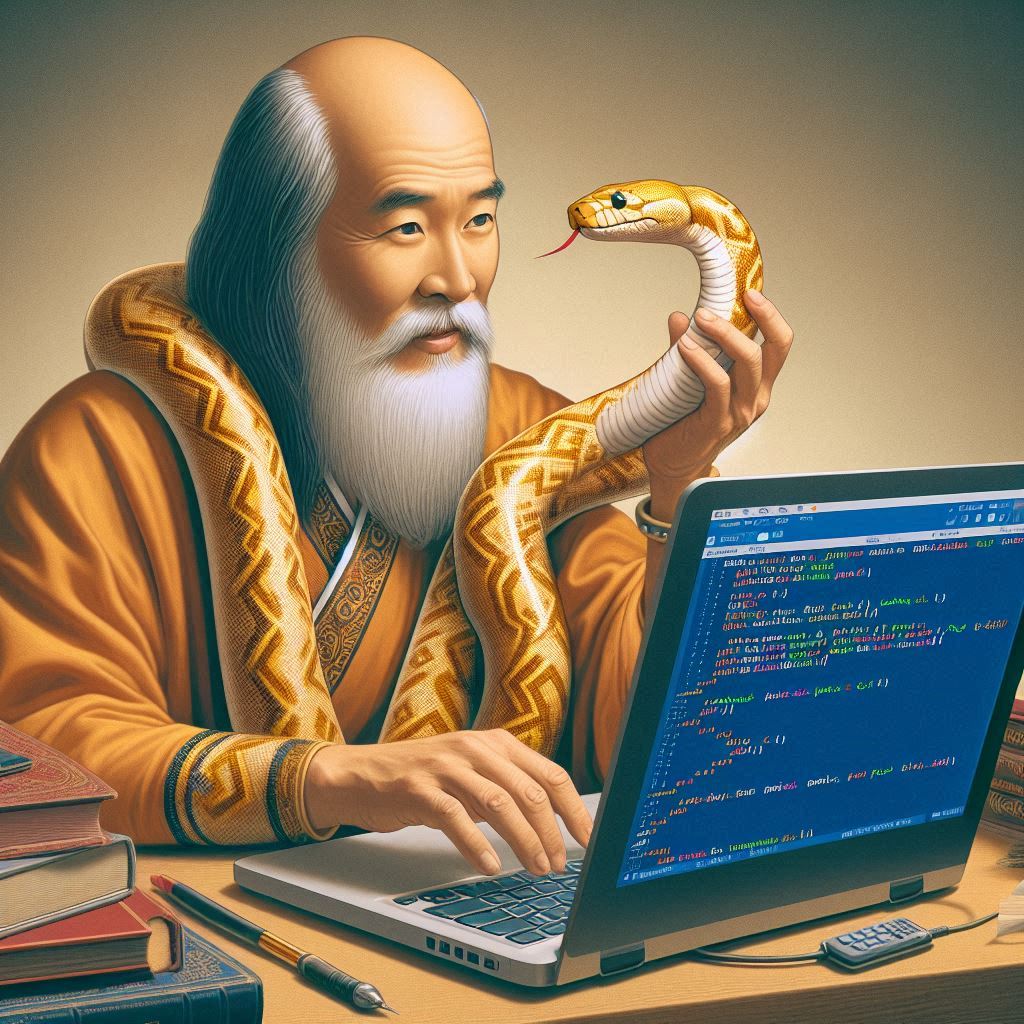
Get Started with Python
- Understand what is Python : https://datartic.com/python-programming-language-layman-series/
- Amazing notes documented at notion : https://sunnybyli.notion.site/Basics-of-Python-5f0c1cea4a264eb3b3c29949790abb62#a55fd20389d749a48c2bd3f92773bce5
Homework 1: Variables and Data Types
- Declare and Print Variables: Declare five variables with different data types (e.g.,
int
,float
,str
,bool
,list
) and print their values using theprint()
function. - Basic Operations: Perform basic arithmetic operations (e.g., addition, subtraction, multiplication, division) using Python and print the results.
- String Manipulation: Use string methods (e.g.,
upper()
,lower()
,strip()
) to manipulate a given string and print the results.
Homework 2: Control Structures
- If-Else Statements: Write a Python program that uses if-else statements to determine whether a given number is even or odd.
- For Loops: Use a for loop to iterate over a list of numbers and print their squares.
- While Loops: Write a Python program that uses a while loop to print the numbers from 1 to 10.
Homework 3: Functions and Modules
- Simple Function: Define a simple function that takes a name as an argument and returns a greeting message.
- Function with Arguments: Define a function that takes two numbers as arguments and returns their sum.
- Importing Modules: Import the
math
module and use its functions (e.g.,sin()
,cos()
) to calculate the sine and cosine of a given angle.
Homework 4: Lists and Tuples
- List Operations: Perform basic list operations (e.g., indexing, slicing, append, extend) and print the results.
- Tuple Operations: Perform basic tuple operations (e.g., indexing, slicing) and print the results.
- List Comprehension: Use list comprehension to create a new list from an existing list.
Projects
Project 1: Calculator Program
Create a Python program that acts as a simple calculator. The program should take in user input for two numbers and an operation (e.g., addition, subtraction, multiplication, division) and print the result.
Project 2: To-Do List App
Create a Python program that allows users to add, remove, and mark tasks as completed in a to-do list. The program should use lists to store the tasks and provide a simple text-based interface.
Project 3: Rock, Paper, Scissors Game
Create a Python program that allows two players to play a game of Rock, Paper, Scissors. The program should use random number generation to determine the winner and provide a simple text-based interface.
These homework and project ideas should help reinforce understanding of the basics of Python programming. Good luck!
- Declare and Print Variables: Declare five variables with different data types (e.g.,
Solutions :